pathlib
--- 物件導向檔案系統路徑¶
在 3.4 版新加入.
原始碼:Lib/pathlib.py
此模組提供代表檔案系統路徑的類別,能適用不同作業系統的語意。路徑類別分成兩種,一種是純路徑 (pure paths),提供沒有 I/O 的單純計算操作,另一種是實體路徑 (concrete paths),繼承自純路徑但也提供 IO 操作。
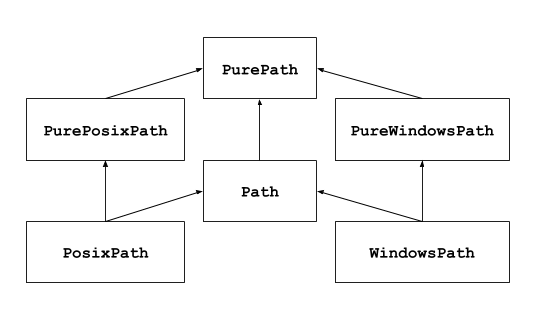
如果你之前從未使用過此模組或不確定哪個類別適合你的任務,那你需要的最有可能是 Path
。它針對程式執行所在的平台實例化一個實體路徑。
純路徑在某些特殊情境下是有用的,例如:
如果你想在 Unix 機器上處理 Windows 路徑(或反過來),你無法在 Unix 上實例化
WindowsPath
,但你可以實例化PureWindowsPath
。你想確保你的程式在操作路徑的時候不會真的存取到 OS。在這個情況下,實例化其中一種純路徑類別可能是有用的,因為它們不會有任何存取 OS 的操作。
也參考
PEP 428:pathlib 模組 -- 物件導向檔案系統路徑。
也參考
針對字串上的底層路徑操作,你也可以使用 os.path
模組。
基本用法¶
匯入主要類別:
>>> from pathlib import Path
列出子目錄:
>>> p = Path('.')
>>> [x for x in p.iterdir() if x.is_dir()]
[PosixPath('.hg'), PosixPath('docs'), PosixPath('dist'),
PosixPath('__pycache__'), PosixPath('build')]
在當前目錄樹下列出 Python 原始碼檔案:
>>> list(p.glob('**/*.py'))
[PosixPath('test_pathlib.py'), PosixPath('setup.py'),
PosixPath('pathlib.py'), PosixPath('docs/conf.py'),
PosixPath('build/lib/pathlib.py')]
瀏覽目錄樹內部:
>>> p = Path('/etc')
>>> q = p / 'init.d' / 'reboot'
>>> q
PosixPath('/etc/init.d/reboot')
>>> q.resolve()
PosixPath('/etc/rc.d/init.d/halt')
查詢路徑屬性:
>>> q.exists()
True
>>> q.is_dir()
False
開啟檔案:
>>> with q.open() as f: f.readline()
...
'#!/bin/bash\n'
純路徑¶
純路徑物件提供處理路徑的操作,實際上不會存取檔案系統。有三種方法可以存取這些類別,我們也稱之為類型 (flavours):
- class pathlib.PurePath(*pathsegments)¶
一個通用的類別,表示系統的路徑類型(實例化時會建立一個
PurePosixPath
或PureWindowsPath
):>>> PurePath('setup.py') # Running on a Unix machine PurePosixPath('setup.py')
pathsegments 中的每個元素可以是以下的其中一種:一個表示路徑片段的字串,或一個物件,它實作了
os.PathLike
介面且其中的__fspath__()
方法會回傳字串,就像是另一個路徑物件:>>> PurePath('foo', 'some/path', 'bar') PurePosixPath('foo/some/path/bar') >>> PurePath(Path('foo'), Path('bar')) PurePosixPath('foo/bar')
當沒有給 pathsegments 的時候,會假設是目前的目錄:
>>> PurePath() PurePosixPath('.')
如果一個片段是絕對路徑,則所有之前的片段會被忽略(類似
os.path.join()
):>>> PurePath('/etc', '/usr', 'lib64') PurePosixPath('/usr/lib64') >>> PureWindowsPath('c:/Windows', 'd:bar') PureWindowsPath('d:bar')
在 Windows 系統上,當遇到具有根目錄的相對路徑片段(例如
r'\foo'
)時,磁碟機 (drive) 部分不會被重置:>>> PureWindowsPath('c:/Windows', '/Program Files') PureWindowsPath('c:/Program Files')
不必要的斜線和單點會被合併,但雙點 (
'..'
) 和前置的雙斜線 ('//'
) 不會被合併,因為這樣會因為各種原因改變路徑的意義(例如符號連結 (symbolic links)、UNC 路徑):>>> PurePath('foo//bar') PurePosixPath('foo/bar') >>> PurePath('//foo/bar') PurePosixPath('//foo/bar') >>> PurePath('foo/./bar') PurePosixPath('foo/bar') >>> PurePath('foo/../bar') PurePosixPath('foo/../bar')
(一個使得
PurePosixPath('foo/../bar')
等同於PurePosixPath('bar')
的單純方法,但如果foo
是指到另一個目錄的符號連結,就會是錯誤的。)純路徑物件實作了
os.PathLike
介面,使得它們可以在任何接受該介面的地方使用。在 3.6 版的變更: 新增了對於
os.PathLike
介面的支援。
- class pathlib.PurePosixPath(*pathsegments)¶
PurePath
的一個子類別,該路徑類型表示非 Windows 檔案系統的路徑:>>> PurePosixPath('/etc') PurePosixPath('/etc')
pathsegments 的指定方式與
PurePath
類似。
- class pathlib.PureWindowsPath(*pathsegments)¶
PurePath
的一個子類別,該路徑類型表示 Windows 檔案系統的路徑,包括 UNC paths:>>> PureWindowsPath('c:/Program Files/') PureWindowsPath('c:/Program Files') >>> PureWindowsPath('//server/share/file') PureWindowsPath('//server/share/file')
pathsegments 的指定方式與
PurePath
類似。
不論你使用的是什麼系統,你都可以實例化這些類別,因為它們不提供任何涉及系統呼叫的操作。
通用屬性¶
路徑物件是不可變 (immutable) 且可雜湊 (hashable) 的。相同類型的路徑物件可以被比較和排序。這些屬性遵守該類型的大小寫語意規則:
>>> PurePosixPath('foo') == PurePosixPath('FOO')
False
>>> PureWindowsPath('foo') == PureWindowsPath('FOO')
True
>>> PureWindowsPath('FOO') in { PureWindowsPath('foo') }
True
>>> PureWindowsPath('C:') < PureWindowsPath('d:')
True
不同類型的路徑物件在比較時視為不相等且無法被排序:
>>> PureWindowsPath('foo') == PurePosixPath('foo')
False
>>> PureWindowsPath('foo') < PurePosixPath('foo')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: '<' not supported between instances of 'PureWindowsPath' and 'PurePosixPath'
運算子¶
斜線運算子 (slash operator) 用於建立子路徑,就像是 os.path.join()
函式一樣。如果引數是絕對路徑,則忽略前一個路徑。在 Windows 系統上,當引數是具有根目錄的相對路徑(例如,r’\foo’
),磁碟機部分不會被重置:
>>> p = PurePath('/etc')
>>> p
PurePosixPath('/etc')
>>> p / 'init.d' / 'apache2'
PurePosixPath('/etc/init.d/apache2')
>>> q = PurePath('bin')
>>> '/usr' / q
PurePosixPath('/usr/bin')
>>> p / '/an_absolute_path'
PurePosixPath('/an_absolute_path')
>>> PureWindowsPath('c:/Windows', '/Program Files')
PureWindowsPath('c:/Program Files')
路徑物件可以被用在任何可以接受實作 os.PathLike
的物件的地方:
>>> import os
>>> p = PurePath('/etc')
>>> os.fspath(p)
'/etc'
路徑的字串表示是原始的檔案系統路徑本身(以原生的形式,例如在 Windows 下是反斜線),你可以將其傳入任何將檔案路徑當作字串傳入的函式:
>>> p = PurePath('/etc')
>>> str(p)
'/etc'
>>> p = PureWindowsPath('c:/Program Files')
>>> str(p)
'c:\\Program Files'
類似地,對路徑呼叫 bytes
會得到原始檔案系統路徑的 bytes 物件,就像使用 os.fsencode()
編碼過的一樣:
>>> bytes(p)
b'/etc'
備註
只建議在 Unix 下呼叫 bytes
。在 Windows 裡,unicode 形式是檔案系統路徑的權威表示方式。
對個別組成的存取¶
可以使用下列屬性來存取路徑的個別「組成」(parts, components):
- PurePath.parts¶
一個可存取路徑的各組成的元組:
>>> p = PurePath('/usr/bin/python3') >>> p.parts ('/', 'usr', 'bin', 'python3') >>> p = PureWindowsPath('c:/Program Files/PSF') >>> p.parts ('c:\\', 'Program Files', 'PSF')
(特別注意磁碟機跟本地根目錄是如何被重新組合成一個單一組成)
方法與屬性¶
純路徑提供以下方法與屬性:
- PurePath.drive¶
若存在則為一個表示磁碟機字母 (drive letter) 或磁碟機名稱 (drive name) 的字串:
>>> PureWindowsPath('c:/Program Files/').drive 'c:' >>> PureWindowsPath('/Program Files/').drive '' >>> PurePosixPath('/etc').drive ''
UNC shares 也被視為磁碟機:
>>> PureWindowsPath('//host/share/foo.txt').drive '\\\\host\\share'
- PurePath.root¶
若存在則為一個表示(本地或全域)根目錄的字串:
>>> PureWindowsPath('c:/Program Files/').root '\\' >>> PureWindowsPath('c:Program Files/').root '' >>> PurePosixPath('/etc').root '/'
UNC shares 都會有一個根目錄:
>>> PureWindowsPath('//host/share').root '\\'
如果路徑以超過兩個連續的斜線開頭,
PurePosixPath
會合併它們:>>> PurePosixPath('//etc').root '//' >>> PurePosixPath('///etc').root '/' >>> PurePosixPath('////etc').root '/'
備註
此行為符合 The Open Group Base Specifications Issue 6,章節 4.11 路徑名稱解析:
「以兩個連續斜線開頭的路徑名稱可以根據實作定義的方式來解讀,儘管如此,開頭超過兩個斜線應該視為單一斜線。」
- PurePath.anchor¶
磁碟機與根目錄的結合:
>>> PureWindowsPath('c:/Program Files/').anchor 'c:\\' >>> PureWindowsPath('c:Program Files/').anchor 'c:' >>> PurePosixPath('/etc').anchor '/' >>> PureWindowsPath('//host/share').anchor '\\\\host\\share\\'
- PurePath.parents¶
一個不可變的序列,為路徑邏輯上的祖先 (logical ancestors) 提供存取:
>>> p = PureWindowsPath('c:/foo/bar/setup.py') >>> p.parents[0] PureWindowsPath('c:/foo/bar') >>> p.parents[1] PureWindowsPath('c:/foo') >>> p.parents[2] PureWindowsPath('c:/')
在 3.10 版的變更: 父序列現在支援 slices 及負的索引值。
- PurePath.parent¶
邏輯上的父路徑:
>>> p = PurePosixPath('/a/b/c/d') >>> p.parent PurePosixPath('/a/b/c')
你不能越過一個 anchor 或空路徑:
>>> p = PurePosixPath('/') >>> p.parent PurePosixPath('/') >>> p = PurePosixPath('.') >>> p.parent PurePosixPath('.')
備註
這是一個純粹字句上的 (lexical) 運算,因此會有以下行為:
>>> p = PurePosixPath('foo/..') >>> p.parent PurePosixPath('foo')
如果你想要沿任意的檔案系統路徑往上走,建議要先呼叫
Path.resolve()
來解析符號連結 (symlink) 及去除其中的”..”
。
- PurePath.name¶
最後的路徑組成 (final path component) 的字串表示,不包含任何磁碟機或根目錄:
>>> PurePosixPath('my/library/setup.py').name 'setup.py'
UNC 磁碟機名稱並沒有算在內:
>>> PureWindowsPath('//some/share/setup.py').name 'setup.py' >>> PureWindowsPath('//some/share').name ''
- PurePath.suffix¶
若存在則為最後的路徑組成的檔案副檔名:
>>> PurePosixPath('my/library/setup.py').suffix '.py' >>> PurePosixPath('my/library.tar.gz').suffix '.gz' >>> PurePosixPath('my/library').suffix ''
- PurePath.suffixes¶
路徑檔案副檔名的串列:
>>> PurePosixPath('my/library.tar.gar').suffixes ['.tar', '.gar'] >>> PurePosixPath('my/library.tar.gz').suffixes ['.tar', '.gz'] >>> PurePosixPath('my/library').suffixes []
- PurePath.stem¶
最後的路徑組成,不包括後綴 (suffix):
>>> PurePosixPath('my/library.tar.gz').stem 'library.tar' >>> PurePosixPath('my/library.tar').stem 'library' >>> PurePosixPath('my/library').stem 'library'
- PurePath.as_posix()¶
回傳一個使用正斜線 (
/
) 的路徑的字串表示:>>> p = PureWindowsPath('c:\\windows') >>> str(p) 'c:\\windows' >>> p.as_posix() 'c:/windows'
- PurePath.as_uri()¶
以
file
URI 來表示一個路徑。如果不是絕對路徑會引發ValueError
。>>> p = PurePosixPath('/etc/passwd') >>> p.as_uri() 'file:///etc/passwd' >>> p = PureWindowsPath('c:/Windows') >>> p.as_uri() 'file:///c:/Windows'
- PurePath.is_absolute()¶
回傳一個路徑是否是絕對路徑。一個路徑被視為絕對路徑的條件是它同時有根目錄及(如果該系統類型允許的話)磁碟機:
>>> PurePosixPath('/a/b').is_absolute() True >>> PurePosixPath('a/b').is_absolute() False >>> PureWindowsPath('c:/a/b').is_absolute() True >>> PureWindowsPath('/a/b').is_absolute() False >>> PureWindowsPath('c:').is_absolute() False >>> PureWindowsPath('//some/share').is_absolute() True
- PurePath.is_relative_to(other)¶
回傳此路徑是否為 other 路徑的相對路徑。
>>> p = PurePath('/etc/passwd') >>> p.is_relative_to('/etc') True >>> p.is_relative_to('/usr') False
在 3.9 版新加入.
自從版本 3.12 後不推薦使用,將會自版本 3.14 中移除。: 額外引數的傳入已棄用;如果有的話,它們會與 other 連接在一起。
- PurePath.is_reserved()¶
對
PureWindowsPath
來說,當路徑在 Windows 下被視為保留的話會回傳True
,否則回傳False
。對PurePosixPath
來說,總是回傳False
。>>> PureWindowsPath('nul').is_reserved() True >>> PurePosixPath('nul').is_reserved() False
在保留路徑上的檔案系統呼叫會神秘地失敗或有意外的效果。
- PurePath.joinpath(*pathsegments)¶
呼叫此方法會依序結合每個所給定的 pathsegments 到路徑上:
>>> PurePosixPath('/etc').joinpath('passwd') PurePosixPath('/etc/passwd') >>> PurePosixPath('/etc').joinpath(PurePosixPath('passwd')) PurePosixPath('/etc/passwd') >>> PurePosixPath('/etc').joinpath('init.d', 'apache2') PurePosixPath('/etc/init.d/apache2') >>> PureWindowsPath('c:').joinpath('/Program Files') PureWindowsPath('c:/Program Files')
- PurePath.match(pattern, *, case_sensitive=None)¶
將路徑與 glob 形式的樣式 (glob-style pattern) 做比對。如果比對成功則回傳
True
,否則回傳False
。如果 pattern 是相對的,則路徑可以是相對或絕對的,而且會從右邊來完成比對:
>>> PurePath('a/b.py').match('*.py') True >>> PurePath('/a/b/c.py').match('b/*.py') True >>> PurePath('/a/b/c.py').match('a/*.py') False
如果 pattern 是絕對的,則路徑必須是絕對的,且整個路徑都要比對到:
>>> PurePath('/a.py').match('/*.py') True >>> PurePath('a/b.py').match('/*.py') False
pattern 可以是另一個路徑物件;這會加速對多個檔案比對相同的樣式:
>>> pattern = PurePath('*.py') >>> PurePath('a/b.py').match(pattern) True
在 3.12 版的變更: 接受一個有實作
os.PathLike
介面的物件。像其它方法一樣,是否區分大小寫會遵循平台的預設行為:
>>> PurePosixPath('b.py').match('*.PY') False >>> PureWindowsPath('b.py').match('*.PY') True
將 case_sensitive 設定成
True
或False
會覆蓋這個行為。在 3.12 版的變更: 新增 case_sensitive 參數。
- PurePath.relative_to(other, walk_up=False)¶
計算這個路徑相對於 other 所表示路徑的版本。如果做不到會引發
ValueError
:>>> p = PurePosixPath('/etc/passwd') >>> p.relative_to('/') PurePosixPath('etc/passwd') >>> p.relative_to('/etc') PurePosixPath('passwd') >>> p.relative_to('/usr') Traceback (most recent call last): File "<stdin>", line 1, in <module> File "pathlib.py", line 941, in relative_to raise ValueError(error_message.format(str(self), str(formatted))) ValueError: '/etc/passwd' is not in the subpath of '/usr' OR one path is relative and the other is absolute.
當 walk_up 是 False(預設值),路徑必須以 other 為開始。當此引數是 True,可能會加入
..
以組成相對路徑。在其他情況下,例如路徑參考到不同的磁碟機,則會引發ValueError
:>>> p.relative_to('/usr', walk_up=True) PurePosixPath('../etc/passwd') >>> p.relative_to('foo', walk_up=True) Traceback (most recent call last): File "<stdin>", line 1, in <module> File "pathlib.py", line 941, in relative_to raise ValueError(error_message.format(str(self), str(formatted))) ValueError: '/etc/passwd' is not on the same drive as 'foo' OR one path is relative and the other is absolute.
警告
這個函式是
PurePath
的一部分且可以在字串上運作。它不會檢查或存取實際的檔案架構。這會影響到 walk_up 選項,因為它假設路徑中沒有符號連結;如果需要解析符號連結的話可以先呼叫resolve()
。在 3.12 版的變更: 加入 walk_up 參數(舊的行為和
walk_up=False
相同)。自從版本 3.12 後不推薦使用,將會自版本 3.14 中移除。: 額外位置引數的傳入已棄用;如果有的話,它們會與 other 連接在一起。
- PurePath.with_name(name)¶
回傳一個修改
name
後的新路徑。如果原始路徑沒有名稱則引發 ValueError:>>> p = PureWindowsPath('c:/Downloads/pathlib.tar.gz') >>> p.with_name('setup.py') PureWindowsPath('c:/Downloads/setup.py') >>> p = PureWindowsPath('c:/') >>> p.with_name('setup.py') Traceback (most recent call last): File "<stdin>", line 1, in <module> File "/home/antoine/cpython/default/Lib/pathlib.py", line 751, in with_name raise ValueError("%r has an empty name" % (self,)) ValueError: PureWindowsPath('c:/') has an empty name
- PurePath.with_stem(stem)¶
回傳一個修改
stem
後的新路徑。如果原始路徑沒有名稱則引發 ValueError:>>> p = PureWindowsPath('c:/Downloads/draft.txt') >>> p.with_stem('final') PureWindowsPath('c:/Downloads/final.txt') >>> p = PureWindowsPath('c:/Downloads/pathlib.tar.gz') >>> p.with_stem('lib') PureWindowsPath('c:/Downloads/lib.gz') >>> p = PureWindowsPath('c:/') >>> p.with_stem('') Traceback (most recent call last): File "<stdin>", line 1, in <module> File "/home/antoine/cpython/default/Lib/pathlib.py", line 861, in with_stem return self.with_name(stem + self.suffix) File "/home/antoine/cpython/default/Lib/pathlib.py", line 851, in with_name raise ValueError("%r has an empty name" % (self,)) ValueError: PureWindowsPath('c:/') has an empty name
在 3.9 版新加入.
- PurePath.with_suffix(suffix)¶
回傳一個修改
suffix
後的新路徑。如果原始路徑沒有後綴,新的 suffix 會附加在後面。如果 suffix 是一個空字串,原來的後綴會被移除:>>> p = PureWindowsPath('c:/Downloads/pathlib.tar.gz') >>> p.with_suffix('.bz2') PureWindowsPath('c:/Downloads/pathlib.tar.bz2') >>> p = PureWindowsPath('README') >>> p.with_suffix('.txt') PureWindowsPath('README.txt') >>> p = PureWindowsPath('README.txt') >>> p.with_suffix('') PureWindowsPath('README')
- PurePath.with_segments(*pathsegments)¶
透過結合給定的 pathsegments 建立一個相同類型的新路徑物件,當一個衍生路徑被建立的時候會呼叫這個方法,例如從
parent
和relative_to()
建立衍生路徑。子類別可以覆寫此方法來傳遞資訊給衍生路徑,例如:from pathlib import PurePosixPath class MyPath(PurePosixPath): def __init__(self, *pathsegments, session_id): super().__init__(*pathsegments) self.session_id = session_id def with_segments(self, *pathsegments): return type(self)(*pathsegments, session_id=self.session_id) etc = MyPath('/etc', session_id=42) hosts = etc / 'hosts' print(hosts.session_id) # 42
在 3.12 版新加入.
Concrete paths¶
Concrete paths are subclasses of the pure path classes. In addition to operations provided by the latter, they also provide methods to do system calls on path objects. There are three ways to instantiate concrete paths:
- class pathlib.Path(*pathsegments)¶
A subclass of
PurePath
, this class represents concrete paths of the system's path flavour (instantiating it creates either aPosixPath
or aWindowsPath
):>>> Path('setup.py') PosixPath('setup.py')
pathsegments 的指定方式與
PurePath
類似。
- class pathlib.PosixPath(*pathsegments)¶
A subclass of
Path
andPurePosixPath
, this class represents concrete non-Windows filesystem paths:>>> PosixPath('/etc') PosixPath('/etc')
pathsegments 的指定方式與
PurePath
類似。
- class pathlib.WindowsPath(*pathsegments)¶
A subclass of
Path
andPureWindowsPath
, this class represents concrete Windows filesystem paths:>>> WindowsPath('c:/Program Files/') WindowsPath('c:/Program Files')
pathsegments 的指定方式與
PurePath
類似。
You can only instantiate the class flavour that corresponds to your system (allowing system calls on non-compatible path flavours could lead to bugs or failures in your application):
>>> import os
>>> os.name
'posix'
>>> Path('setup.py')
PosixPath('setup.py')
>>> PosixPath('setup.py')
PosixPath('setup.py')
>>> WindowsPath('setup.py')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "pathlib.py", line 798, in __new__
% (cls.__name__,))
NotImplementedError: cannot instantiate 'WindowsPath' on your system
Methods¶
Concrete paths provide the following methods in addition to pure paths
methods. Many of these methods can raise an OSError
if a system
call fails (for example because the path doesn't exist).
在 3.8 版的變更: exists()
, is_dir()
, is_file()
,
is_mount()
, is_symlink()
,
is_block_device()
, is_char_device()
,
is_fifo()
, is_socket()
now return False
instead of raising an exception for paths that contain characters
unrepresentable at the OS level.
- classmethod Path.cwd()¶
Return a new path object representing the current directory (as returned by
os.getcwd()
):>>> Path.cwd() PosixPath('/home/antoine/pathlib')
- classmethod Path.home()¶
Return a new path object representing the user's home directory (as returned by
os.path.expanduser()
with~
construct). If the home directory can't be resolved,RuntimeError
is raised.>>> Path.home() PosixPath('/home/antoine')
在 3.5 版新加入.
- Path.stat(*, follow_symlinks=True)¶
Return a
os.stat_result
object containing information about this path, likeos.stat()
. The result is looked up at each call to this method.This method normally follows symlinks; to stat a symlink add the argument
follow_symlinks=False
, or uselstat()
.>>> p = Path('setup.py') >>> p.stat().st_size 956 >>> p.stat().st_mtime 1327883547.852554
在 3.10 版的變更: 新增 follow_symlinks 參數。
- Path.chmod(mode, *, follow_symlinks=True)¶
Change the file mode and permissions, like
os.chmod()
.This method normally follows symlinks. Some Unix flavours support changing permissions on the symlink itself; on these platforms you may add the argument
follow_symlinks=False
, or uselchmod()
.>>> p = Path('setup.py') >>> p.stat().st_mode 33277 >>> p.chmod(0o444) >>> p.stat().st_mode 33060
在 3.10 版的變更: 新增 follow_symlinks 參數。
- Path.exists(*, follow_symlinks=True)¶
Return
True
if the path points to an existing file or directory.This method normally follows symlinks; to check if a symlink exists, add the argument
follow_symlinks=False
.>>> Path('.').exists() True >>> Path('setup.py').exists() True >>> Path('/etc').exists() True >>> Path('nonexistentfile').exists() False
在 3.12 版的變更: 新增 follow_symlinks 參數。
- Path.expanduser()¶
Return a new path with expanded
~
and~user
constructs, as returned byos.path.expanduser()
. If a home directory can't be resolved,RuntimeError
is raised.>>> p = PosixPath('~/films/Monty Python') >>> p.expanduser() PosixPath('/home/eric/films/Monty Python')
在 3.5 版新加入.
- Path.glob(pattern, *, case_sensitive=None)¶
Glob the given relative pattern in the directory represented by this path, yielding all matching files (of any kind):
>>> sorted(Path('.').glob('*.py')) [PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')] >>> sorted(Path('.').glob('*/*.py')) [PosixPath('docs/conf.py')]
Patterns are the same as for
fnmatch
, with the addition of "**
" which means "this directory and all subdirectories, recursively". In other words, it enables recursive globbing:>>> sorted(Path('.').glob('**/*.py')) [PosixPath('build/lib/pathlib.py'), PosixPath('docs/conf.py'), PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')]
By default, or when the case_sensitive keyword-only argument is set to
None
, this method matches paths using platform-specific casing rules: typically, case-sensitive on POSIX, and case-insensitive on Windows. Set case_sensitive toTrue
orFalse
to override this behaviour.備註
Using the "
**
" pattern in large directory trees may consume an inordinate amount of time.引發一個附帶引數
self
、pattern
的稽核事件pathlib.Path.glob
。在 3.11 版的變更: Return only directories if pattern ends with a pathname components separator (
sep
oraltsep
).在 3.12 版的變更: 新增 case_sensitive 參數。
- Path.group()¶
Return the name of the group owning the file.
KeyError
is raised if the file's gid isn't found in the system database.
- Path.is_dir()¶
Return
True
if the path points to a directory (or a symbolic link pointing to a directory),False
if it points to another kind of file.False
is also returned if the path doesn't exist or is a broken symlink; other errors (such as permission errors) are propagated.
- Path.is_file()¶
Return
True
if the path points to a regular file (or a symbolic link pointing to a regular file),False
if it points to another kind of file.False
is also returned if the path doesn't exist or is a broken symlink; other errors (such as permission errors) are propagated.
- Path.is_junction()¶
Return
True
if the path points to a junction, andFalse
for any other type of file. Currently only Windows supports junctions.在 3.12 版新加入.
- Path.is_mount()¶
Return
True
if the path is a mount point: a point in a file system where a different file system has been mounted. On POSIX, the function checks whether path's parent,path/..
, is on a different device than path, or whetherpath/..
and path point to the same i-node on the same device --- this should detect mount points for all Unix and POSIX variants. On Windows, a mount point is considered to be a drive letter root (e.g.c:\
), a UNC share (e.g.\\server\share
), or a mounted filesystem directory.在 3.7 版新加入.
在 3.12 版的變更: Windows support was added.
- Path.is_symlink()¶
Return
True
if the path points to a symbolic link,False
otherwise.False
is also returned if the path doesn't exist; other errors (such as permission errors) are propagated.
- Path.is_socket()¶
Return
True
if the path points to a Unix socket (or a symbolic link pointing to a Unix socket),False
if it points to another kind of file.False
is also returned if the path doesn't exist or is a broken symlink; other errors (such as permission errors) are propagated.
- Path.is_fifo()¶
Return
True
if the path points to a FIFO (or a symbolic link pointing to a FIFO),False
if it points to another kind of file.False
is also returned if the path doesn't exist or is a broken symlink; other errors (such as permission errors) are propagated.
- Path.is_block_device()¶
Return
True
if the path points to a block device (or a symbolic link pointing to a block device),False
if it points to another kind of file.False
is also returned if the path doesn't exist or is a broken symlink; other errors (such as permission errors) are propagated.
- Path.is_char_device()¶
Return
True
if the path points to a character device (or a symbolic link pointing to a character device),False
if it points to another kind of file.False
is also returned if the path doesn't exist or is a broken symlink; other errors (such as permission errors) are propagated.
- Path.iterdir()¶
When the path points to a directory, yield path objects of the directory contents:
>>> p = Path('docs') >>> for child in p.iterdir(): child ... PosixPath('docs/conf.py') PosixPath('docs/_templates') PosixPath('docs/make.bat') PosixPath('docs/index.rst') PosixPath('docs/_build') PosixPath('docs/_static') PosixPath('docs/Makefile')
The children are yielded in arbitrary order, and the special entries
'.'
and'..'
are not included. If a file is removed from or added to the directory after creating the iterator, whether a path object for that file be included is unspecified.
- Path.walk(top_down=True, on_error=None, follow_symlinks=False)¶
Generate the file names in a directory tree by walking the tree either top-down or bottom-up.
For each directory in the directory tree rooted at self (including self but excluding '.' and '..'), the method yields a 3-tuple of
(dirpath, dirnames, filenames)
.dirpath is a
Path
to the directory currently being walked, dirnames is a list of strings for the names of subdirectories in dirpath (excluding'.'
and'..'
), and filenames is a list of strings for the names of the non-directory files in dirpath. To get a full path (which begins with self) to a file or directory in dirpath, dodirpath / name
. Whether or not the lists are sorted is file system-dependent.If the optional argument top_down is true (which is the default), the triple for a directory is generated before the triples for any of its subdirectories (directories are walked top-down). If top_down is false, the triple for a directory is generated after the triples for all of its subdirectories (directories are walked bottom-up). No matter the value of top_down, the list of subdirectories is retrieved before the triples for the directory and its subdirectories are walked.
When top_down is true, the caller can modify the dirnames list in-place (for example, using
del
or slice assignment), andPath.walk()
will only recurse into the subdirectories whose names remain in dirnames. This can be used to prune the search, or to impose a specific order of visiting, or even to informPath.walk()
about directories the caller creates or renames before it resumesPath.walk()
again. Modifying dirnames when top_down is false has no effect on the behavior ofPath.walk()
since the directories in dirnames have already been generated by the time dirnames is yielded to the caller.By default, errors from
os.scandir()
are ignored. If the optional argument on_error is specified, it should be a callable; it will be called with one argument, anOSError
instance. The callable can handle the error to continue the walk or re-raise it to stop the walk. Note that the filename is available as thefilename
attribute of the exception object.By default,
Path.walk()
does not follow symbolic links, and instead adds them to the filenames list. Set follow_symlinks to true to resolve symlinks and place them in dirnames and filenames as appropriate for their targets, and consequently visit directories pointed to by symlinks (where supported).備註
Be aware that setting follow_symlinks to true can lead to infinite recursion if a link points to a parent directory of itself.
Path.walk()
does not keep track of the directories it has already visited.備註
Path.walk()
assumes the directories it walks are not modified during execution. For example, if a directory from dirnames has been replaced with a symlink and follow_symlinks is false,Path.walk()
will still try to descend into it. To prevent such behavior, remove directories from dirnames as appropriate.備註
Unlike
os.walk()
,Path.walk()
lists symlinks to directories in filenames if follow_symlinks is false.This example displays the number of bytes used by all files in each directory, while ignoring
__pycache__
directories:from pathlib import Path for root, dirs, files in Path("cpython/Lib/concurrent").walk(on_error=print): print( root, "consumes", sum((root / file).stat().st_size for file in files), "bytes in", len(files), "non-directory files" ) if '__pycache__' in dirs: dirs.remove('__pycache__')
This next example is a simple implementation of
shutil.rmtree()
. Walking the tree bottom-up is essential asrmdir()
doesn't allow deleting a directory before it is empty:# Delete everything reachable from the directory "top". # CAUTION: This is dangerous! For example, if top == Path('/'), # it could delete all of your files. for root, dirs, files in top.walk(top_down=False): for name in files: (root / name).unlink() for name in dirs: (root / name).rmdir()
在 3.12 版新加入.
- Path.lchmod(mode)¶
Like
Path.chmod()
but, if the path points to a symbolic link, the symbolic link's mode is changed rather than its target's.
- Path.lstat()¶
Like
Path.stat()
but, if the path points to a symbolic link, return the symbolic link's information rather than its target's.
- Path.mkdir(mode=0o777, parents=False, exist_ok=False)¶
Create a new directory at this given path. If mode is given, it is combined with the process'
umask
value to determine the file mode and access flags. If the path already exists,FileExistsError
is raised.If parents is true, any missing parents of this path are created as needed; they are created with the default permissions without taking mode into account (mimicking the POSIX
mkdir -p
command).If parents is false (the default), a missing parent raises
FileNotFoundError
.If exist_ok is false (the default),
FileExistsError
is raised if the target directory already exists.If exist_ok is true,
FileExistsError
exceptions will be ignored (same behavior as the POSIXmkdir -p
command), but only if the last path component is not an existing non-directory file.在 3.5 版的變更: 新增 exist_ok 參數。
- Path.open(mode='r', buffering=-1, encoding=None, errors=None, newline=None)¶
Open the file pointed to by the path, like the built-in
open()
function does:>>> p = Path('setup.py') >>> with p.open() as f: ... f.readline() ... '#!/usr/bin/env python3\n'
- Path.read_bytes()¶
將指向檔案的二進為內容以一個位元組 (bytes) 物件回傳:
>>> p = Path('my_binary_file') >>> p.write_bytes(b'Binary file contents') 20 >>> p.read_bytes() b'Binary file contents'
在 3.5 版新加入.
- Path.read_text(encoding=None, errors=None)¶
將指向檔案的解碼內容以字串形式回傳:
>>> p = Path('my_text_file') >>> p.write_text('Text file contents') 18 >>> p.read_text() 'Text file contents'
該檔案被打開並且隨後關閉。選填參數的含義與
open()
函數中的相同。在 3.5 版新加入.
- Path.readlink()¶
回傳符號連結指向的路徑(如
os.readlink()
的回傳值):>>> p = Path('mylink') >>> p.symlink_to('setup.py') >>> p.readlink() PosixPath('setup.py')
在 3.9 版新加入.
- Path.rename(target)¶
將此檔案或目錄重新命名為所提供的 target ,並回傳一個新的路徑 (Path) 物件指向該 target 。在 Unix 系統上,若 target 存在且為一個檔案,若使用者有權限,則會在不顯示訊息的情況下進行取代。在 Windows 系統上,若 target 存在,則會引發
FileExistsError
錯誤。target 可以是字串或另一個路徑物件:>>> p = Path('foo') >>> p.open('w').write('some text') 9 >>> target = Path('bar') >>> p.rename(target) PosixPath('bar') >>> target.open().read() 'some text'
目標路徑可以是絕對路徑或相對路徑。相對路徑會相對於當前的工作目錄進行解釋,not 相對於路徑物件所在的目錄。
此功能是使用
os.rename()
實現的,並提供相同的保證。在 3.8 版的變更: 新增了回傳值,回傳新的路徑 (Path) 物件。
- Path.replace(target)¶
將此檔案或目錄重新命名為給定的 target ,並回傳一個指向 target 的新路徑物件。如果 target 指向一個現有的檔案或空目錄,它將被無條件地取代。
目標路徑可以是絕對路徑或相對路徑。相對路徑會相對於當前的工作目錄進行解釋,not 相對於路徑物件所在的目錄。
在 3.8 版的變更: 新增了回傳值,回傳新的路徑 (Path) 物件。
- Path.absolute()¶
使路徑成為絕對路徑,不進行標準化或解析符號連結。 回傳一個新的路徑物件:
>>> p = Path('tests') >>> p PosixPath('tests') >>> p.absolute() PosixPath('/home/antoine/pathlib/tests')
- Path.resolve(strict=False)¶
將路徑轉換為絕對路徑,解析所有符號連結。回傳一個新的路徑物件:
>>> p = Path() >>> p PosixPath('.') >>> p.resolve() PosixPath('/home/antoine/pathlib')
同時也會消除 "
..
" 的路徑組件(這是唯一的方法):>>> p = Path('docs/../setup.py') >>> p.resolve() PosixPath('/home/antoine/pathlib/setup.py')
如果路徑不存在且 strict 為
True
, 則引發FileNotFoundError
。如果 strict 為False
, 則將盡可能解析該路徑,並將任何剩餘部分追加到路徑中,而不檢查其是否存在。 如果在解析過程中遇到無窮迴圈,則引發RuntimeError
。在 3.6 版的變更: 新增 strict 參數(在 3.6 版本之前的行為是嚴格的)。
- Path.rglob(pattern, *, case_sensitive=None)¶
遞迴地 glob 給定的相對 pattern。這相當於在給定的相對 pattern 前面加上 "
**/
" 並呼叫Path.glob()
,其中 patterns 和fnmatch
相同:>>> sorted(Path().rglob("*.py")) [PosixPath('build/lib/pathlib.py'), PosixPath('docs/conf.py'), PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')]
By default, or when the case_sensitive keyword-only argument is set to
None
, this method matches paths using platform-specific casing rules: typically, case-sensitive on POSIX, and case-insensitive on Windows. Set case_sensitive toTrue
orFalse
to override this behaviour.引發一個附帶引數
self
、pattern
的稽核事件pathlib.Path.rglob
。在 3.11 版的變更: Return only directories if pattern ends with a pathname components separator (
sep
oraltsep
).在 3.12 版的變更: 新增 case_sensitive 參數。
- Path.rmdir()¶
移除此目錄。該目錄必須為空。
- Path.samefile(other_path)¶
回傳此路徑是否指向與 other_path 相同的檔案,other_path 可以是路徑 (Path) 物件或字串。其語義類似於
os.path.samefile()
和os.path.samestat()
。若任何一個檔案因為某些原因無法存取,則引發
OSError
。>>> p = Path('spam') >>> q = Path('eggs') >>> p.samefile(q) False >>> p.samefile('spam') True
在 3.5 版新加入.
- Path.symlink_to(target, target_is_directory=False)¶
使這個路徑為一個 target 的符號連結。如果在 Windows 的連結目標是資料夾,target_is_directory 應該為 true(預設為
False
)。如果在 POSIX 的連結目標是資料夾,target_is_directory 的數值會被省略。>>> p = Path('mylink') >>> p.symlink_to('setup.py') >>> p.resolve() PosixPath('/home/antoine/pathlib/setup.py') >>> p.stat().st_size 956 >>> p.lstat().st_size 8
備註
引數的順序 (link, target) 和
os.symlink()
相反。
- Path.hardlink_to(target)¶
使這個路徑為與 target 相同檔案的一個硬連結 (hard link)。
備註
引數的順序 (link, target) 和
os.link()
相反。在 3.10 版新加入.
- Path.touch(mode=0o666, exist_ok=True)¶
根據給定路徑來建立一個檔案。如果 mode 有給定,它會與行程的
umask
值結合,以確定檔案模式和存取旗標。當檔案已經存在時,若 exist_ok 為 true 則函式不會失敗(其變更時間會被更新為當下時間),否則FileExistsError
會被引發。
- Path.unlink(missing_ok=False)¶
移除這個檔案或符號連結。如果路徑指到資料夾,請改用
Path.rmdir()
。如果 missing_ok 是 false(預設值),
FileNotFoundError
會在路徑不存在時被引發。如果 missing_ok 為 true,
FileNotFoundError
例外會被忽略(行為與 POSIXrm -f
指令相同)。在 3.8 版的變更: 新增 missing_ok 參數。
- Path.write_bytes(data)¶
以位元組模式開啟指到的檔案,將 data 寫到檔案,並關閉檔案:: :
>>> p = Path('my_binary_file') >>> p.write_bytes(b'Binary file contents') 20 >>> p.read_bytes() b'Binary file contents'
一個名稱相同的已存在檔案會被覆寫。
在 3.5 版新加入.
Correspondence to tools in the os
module¶
Below is a table mapping various os
functions to their corresponding
PurePath
/Path
equivalent.
備註
Not all pairs of functions/methods below are equivalent. Some of them,
despite having some overlapping use-cases, have different semantics. They
include os.path.abspath()
and Path.absolute()
,
os.path.relpath()
and PurePath.relative_to()
.
註解